Chapter Thirteen
Atari Graphics
This chapter and the one that follows are the real payoffs in this book on Atari assembly language. In this chapter and the next, you'll learn how to use assembly language to:
- Custom design your own screen displays, intermixing text, graphics and color in any way you choose.
- Scroll text and graphics on your computer screen.
- Create and use custom designed character sets.
- Use game controllers in assembly language programs.
- Use Atari player-missile graphics to create arcade style action on your computer screen.
To accomplish all of these things, you're going to have to write some fairly complex assembly language routines, but once you've typed and saved them, you'll find that there are many, may ways to use them. By the time you've finished this book, you'll discover (I hope) that you've become a pretty advanced assembly language programmer.
The first program in this chapter will enable you to create a title screen that you can use with any homemade program you like, and it will be a real eye catcher, too! It will display three different sizes of type on your computer screen, with each size of type displayed in a different color, against a background of still another color. And, as they say in those TV mail order ads, there's more. In the next (and last) chapter of this book, you'll learn how to use fine scrolling to animate your title screen. Then , as an extra bonus, you'll learn how to use game controllers and player-missile graphics in Atari assembly language programs. First, though, we'll have to take a brief look at some of the graphics related features of your Atari computer, and at the way it generates its screen display.
The Antic Chip
Atari computer use a much more sophisticated, and much more powerful technique for generating screen displays then most microcomputers do. In most microcomputer, you'll find just one block of RAM in which you can store data that is to appear on the computer's screen, in other words, one block of RAM that's dedicated to screen memory. Within that block of RAM, each letter or symbol that appears on the screen will e assigned one memory location. When the value of that memory location is changed, the text or graphics display in the screen location that corresponds to that memory location will also change. And that's about all you have to know to understand the graphics and text displays of most computer systems.
Atari graphics, as we just said, are more sophisticated than that and just a bit more complicated as well. Atari computer use two special chips, an ANTIC chip and a GTIA chip, to generate their graphics displays. One of these chips, the ANTIC, is a real microprocessor; it is designed to be used with a special instruction set, and a special kind of program called a display list. So, to create graphics using the ANTIC chip, you have to know how to use the instruction set to design display lists for your Atari. It also helps to have a rudimentary knowledge about how a television set works. So here goes:
Scan Lines
The picture on a television screen, as you may know, is made up of tiny horizontal lines – 262 lines, to be exact. And each of these horizontal lines is called a scan line.
As you may also know, these scan lines are produced by an electron gun behind your television set's picture tube. This electronic pistol fires electrons at the phosphor coating inside the TV picture tube in what is know as a raster scan pattern, a zigzag pattern that begins at the upper left-hand corner of the screen and ends in the bottom right-hand corner.
Since there are 262 horizontal scan lines on a video tube, the complete 262 line display on your TV screen is replaced by a completely new display 60 times each second. Between each of these lightning fast scenery changes, there is an extremely brief interval called a vertical blank period, in which the whole screen goes blank.
Because of a picture tube design technique called overscan, however, not all of the 262 scan lines that are available for a TV picture appear on the screen; some fall off the edge and are therefore never seen. So programs used to generate video displays for computer don't usually make use of all of those lines. Your Atari, for example, uses only 192 of the 262 scan lines that are available.
Dot Matrix Characters
If you look at a computer generated text display on a TV screen, you may also notice that each text character on the screen is made up of tiny dots. And if you could look closely enough at the text screen generated by your Atari while your computer is in its normal 40 column by 24 line text mode, you'd be able to see that each letter on the screen is made up of 64 dots, arranged in a matrix 8 dots wide and 8 dots high.
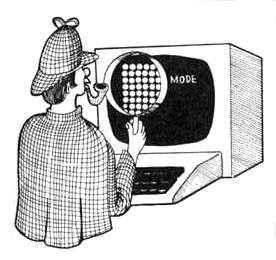
Mode Lines
Your Atari computer has four different text modes. Each of these modes produces letters of a different size. But no matter how big the letters on your screen are, each line of text in an Atari display is called a mode line. In your Atari's normal 40 column by 24 line text mode, the mode referred to in Atari BASIC as Graphics 0, each letter in a mode line is eight dots high, and each of those dots equates to one scan line. In BASIC's Graphics 0 mode, therefore, one mode line is equal to eight scan lines.
Atari BASC supports two other text modes: the Graphics 1 mode, in which the characters on the screen are the same height as Graphics 0 character but twice as wide, and the Graphics 2 mode, in which the characters are twice as high and twice as wide as standard Graphics 0 characters. When your computer is in its Graphics 1 mode, each mode line is made up of eight scan lines the same number of scan lines used in a mode line in Graphics 0. When your Atari is in its Graphics 2 mode however, each mode line equals 16 scan lines.
Antic Mode
In assembly language, there is also another text mode, called ANTIC Mode 3, that is not supported by BASIC. In ANTIC Mode 3, each mode line is made up of 10 scan lines. You can find out more about ANTIC Mode 3 by reading the Atari Programmer's Manual, De Re Atari, or by consulting The Atari 400/800 Technical Reference Notes published by Atari.
In addition to their four text modes, Atari computers have numerous graphics modes; either 10 or 13 of them, depending upon what kind of graphics hardware came installed in your Atari. (The number of graphics modes offered by Atari computer vary, since older Ataris have a graphics chip called CTIA, while newer models come with a new GTIA chip installed.) In non-text graphics modes, the number of scan lines per mode line can range from one (in high resolution graphics) to eight (in low resolution graphics). The number of colors available also differs from graphics mode to graphics mode.
In The Mode
Here is a table of the graphics modes available to Atari assembly language programmers. Sharp-eyed readers will notice that there are differences between the ANTIC designations and the BASIC designations of these modes, and that assembly language supports more modes than Atari BASIC does. The table doesn't include the special modes available to owners of GTIA chips, since this book is for all Atari Home Computers, and program that use those modes won't work properly on all Atari computes. If you want to use them anyway, you can find out how in the assembly language programming guide, De Re Atari.
Atari Text And Graphics Modes
ANTIC BASIC Scan Lines No. Of Mode Mode Per Mode Line Colors 2 0 8 2 3 None 10 2 4 None 8 4 5 None 16 4 6 1 8 5 7 2 16 5 8 3 8 4 9 4 4 2 A 5 4 4 B 6 2 2 C None 1 2 D 7 2 4 E None 1 4 F 8 1 2
Customizing Your Atari's Screen Display
Two steps are needed to custom design an Atari screen display. First you have to create a special kind of program called a display list. Then you have to write a program that will tell your computer how to use the display list you have designed. While doing this be aware that a display list may not cross a 1K boundary and a screen display memory may not cross a 4K boundary with out special handling.
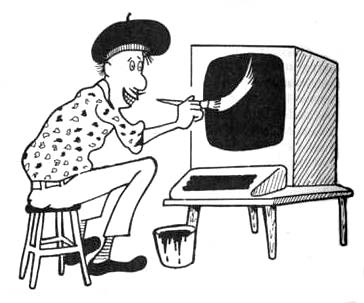
In a moment, we'll talk about how to write a display list program. First, though, let's take a look at the display list that the ANTIC program will refer to. A display list is made up of a series of one byte instructions that can be placed almost anywhere in your computer's available RAM. To get an idea of what a display list looks like, you can use your assembler's debugging utility to examine the display list that your computer uses when it's in it s Graphics 0 text mode.
When you turn on your computer, it automatically goes into Graphics 0 mode, and the address of the display list which it uses to generate that mode is always stored in two locations: memory address $230 and $231. Memory address $230 always holds the low byte of the stating address of the display list that your computer is using, and memory address $231 always hold the high byte of the display list's starting address. So, once you know the contents of these two addresses, you'll be able to locate the display list that your computer is currently using. Once you locate your computer's Graphics 0 display list, you'll find that it looks something like this:
70 70 70 42 20 7C 02 02 02 02 02 02 02 02 02 02 02 02 02 02 02 02 02 02 02 02 02 02 02 41 E0 7B
As you can see, a display list is just that: a list, not a program. To use a display list, a separate program is needed. You'll get a chance to take a look at such a program in a moment. But first, let's examine this sample display list byte by byte.
Bytes 1 - 3
$70 $70 $70
Each byte in a display list has a specific meaning to the Atari ANTIC chip. And within each byte, each nibble (that is each hexadecimal digit) also has a specific meaning. For example, each of the first three bytes – each of the three $70s at the beginning of the list – tells the ANTIC chip to display one blank mode line (in BASIC Graphics 0, eight blank scan lines). A standard Graphics 0 display always begins with three “skip mode line” instructions (in ANTIC language, three $70s) in order to overcome the overscan characteristics of TV tubs and make sure that the whole screen display called for in the display list winds up visible on the screen.
Bytes 4 - 6
$42 $20 $7C
The first actual display byte in our sample display list ($42) is what is known as a Load Memory Scan (LMS) command. The first display byte in a display list, that is, the first byte after all necessary blank lines have been taken care of, is always an LMS command. And a load memory scan command is always a three-byte instruction. In the display list we are now examining, the load memory scan instruction is made up of the three bytes: “$42 $20 $7C.” The first nibble in this instruction, the digit 4, alerts ANTIC that what follows is going to be an LMS instruction. The second nibble in the LMS instruction, the digit 2, tells ANTIC to display an ANTIC Mode 2 line. Consult the table on graphics modes presented a few paragraphs back, and you'll see that in ANTIC language, Mode 2 is the same as BASIC Mode 0. The next two bytes of the LMS command, the bytes $20 $7C, provide ANTIC with the address at which screen memory will begin. ANTIC interprets these two bytes low byte first, in standard 6502 fashion. When ANTIC encounters the LMS instruction $42 $20 $7C, therefore, the first byte displayed on your Atari's video screen will be whatever byte is stored in memory address $7C20.
When you write a display list, you can put your screen memory in just about any convenient and available block of RAM. And you can fill that RAM up with whatever you like: ATASCII codes that equate to text, display screens drawn with the help of a graphics program, or character graphics created with a graphics generator program. Once you have a display created, address in the two bytes that follow your display list's LMS command. You'll have an opportunity to see how this technique works in the sample program at the end of this chapter.
Bytes 7 - 29
The byte $02, repeated 23 times
As explained above, the first LMS command in a display list tells ANTIC two things: the address at which screen memory begins, and the graphics mode to use to display the first mode line of text or data that will be found starting at that address. After ANTIC has been presented with this information, it must be told what graphics mode to use to display each subsequent mode line that will be displayed on the screen. In the display list which we are now examining, every mode line on the screen is an ANTIC Mode 2 line. Therefore the next 23 instructions in this display list are all the same: Each tells ANTIC that the next line on the screen will be an ANTIC Mode 2 line.
What would happen, you may ask, if all of these 23 instructions were not the same? Well, if they were not the same, then more than one graphics mode could be displayed on the screen simultaneously. Text of various sizes could be displayed on the same screen, and text and graphics modes could be intermixed as desired. This is a very powerful and quite unusual capability of Atari computers. You'll get a chance to see exactly how it works before we finish this chapter.
Bytes 30 - 32
$41 $E0 $7B
Every display list must end with a three-byte command called a JVB (Jump on Vertical Blank) instruction. The first byte in a VB instruction is always the value $41. The next two bytes always combine to form a jump address. The destination of the jump is always the beginning of the display list in which the jump is contained. As it happens, the display list we're now looking at starts at memory address $7BE0. So that's the address that follows the JVB instruction $41 in a display list, it jumps back to the beginning of the display list, waits for the next vertical blank period between raster scan displays, and then jumps to the address of the beginning of the display list, what the JVB instruction really does is generate the display list again.
Running a Display List
As we've pointed out, a display list can be placed in almost any convenient and available spot in your computer's memory. Screen memory can be placed just about anywhere in RAM, too. Once you've created a display list and a block of data to be used as screen memory, all you have to do to put your custom designed display on your TV screen is write a simple little assembly language program that tells your computer's operating system where your display list is. To direct your computer to your custom display list, all you have to do is store new values into a pair of OS memory locations known as “shadow” locations. Shadow addresses are used often in Atari programming, so I might as well explain right now what they are.
In your computer's memory, there are some very useful hardware registers that cannot normally be accessed by user-written programs. But sixty times per second, the data in each of these memory locations is updated. During this updating process, the value stored in each of these registers is replaced by data that has been stored in a corresponding shadow register. And shadow registers are in user-accessible RAM. So, by changing the value in a shadow register, you can also change the value of its corresponding hardware register. For most intents and purposes, therefore, a shadow register works just about like any other OS register that's situated in RAM. Three shadow addresses that are often used in display list programs are $22F, $230, and $231. Address $22F is an Atari OS memory location called SDMCTL (Shadow, Direct Memory Access Control). Addresses $230 and $231 are OS locations called SDLSTL (Shadow, Display List Pointer – Low) and SDLSTH (Shadow, Display List Pointer – High). To write a program that will put a custom display list on your Atari's screen, all you have to do is follow these three steps:
- Turn you computer's ANTIC chip off by storing a zero in $22F (SDMCTL).
- Store the starting address of your custom display list in $230 and $231 (SDLSTL and SDLSTH).
- Turn your computer's ANTIC chip on again by storing the value $22 in $22F (SDMCTL).
Doing It
Now that you know how all of those things are done, we're ready to do them. Here is a customized display list along with a program that will run it. If you wish, you can type it into your Atari computer, save it on a disk, and run it right now:
A CUSTOMIZED SCREEN DISPLAY
10 ; 20 ;HELLO SCREEN 30 ; 40 *=$3000 50 JMP INIT 60 ; 70 SDMCTL=$022F 80 ; 90 SDLSTL=$0230 0100 SDLSTH=$0231 0110 ; 0120 COLOR0=$02C4; OS COLOR REGISTERS 0130 COLOR1=$02C5 0140 COLOR2=$02C6 0150 COLOR3=$02C7 0160 COLOR4=$02C8 0170 ; 0180 ;DISPLAY LIST DATA 0190 ; 0200 START 0210 ; 0220 LINE1 .SBYTE " PRESENTING " 0230 LINE2 .SBYTE " the big program " 0240 LINE3 .SBYTE " By (your name) " 0250 ; 0260 LINE4 .SBYTE " PLEASE STAND BY " 0270 ; 0280 ;DISPLAY LIST 0290 ; 0300 HLIST 0310 .BYTE $70,$70,$70; 3 BLANK LINES 0320 .BYTE $70, $70, $70, $70,$70; MORE BLANK LINES 0330 .BYTE $46; LSM, ANTIC MODE 6 (BASIC MODE 2) 0340 .WORD LINE1; (TEXT LINE: "PRESENTING...") 0350 .BYTE $70,$70,$70,$70,$47; LMS, ANTIC MODE 7 0360 .WORD LINE2; (TEXT LINE: "THE BIG PROGRAM") 0370 .BYTE $70,$42; (LMS, ANTIC MODE 2 [GR.0]) 0380 .WORD LINE3;(TEXT LINE: "By [Your Name]") 0390 .BYTE $70,$70,$70,$70,$46;LMS, ANTIC MODE 6 0400 .WORD LINE4; (TEXT LINE: "PLEASE STAND BY") 0410 .BYTE $70,$70,$70,$70,$70; 5 BLANK LINES 0420 .BYTE $41; JVB INSTRUCTION 0430 .WORD HLIST; TO JUMP BACK TO START OF LIST 0440 ; 0450 ;RUN PROGRAM 0460 ; 0470 INIT NOP; SWITCHING COLOR REGISTERS FOR NICELY COLORED DISPLAY 0480 LDA COLOR3 0490 STA COLOR1 0500 LDA COLOR4 0510 STA COLOR2 0520 ; NOW WE'LL RUN THE PROGRAM 0530 LDA #0 0540 STA SDMCTL; TURN ANTIC OFF FOR A MOMENT ... 0550 LDA #HLIST&; WHILE WE STORE OUR NEW LIST'S ADDRESS 0560 STA SDLSTL; IN THE OS DISPLAY POINTER. 0570 LDA # HLIST/256; NOW FOR THE HIGH BYTE. 0580 STA SDLSTH; NOW ANTIC WILL KNOW OUR NEW ADDRESS 0590 LDA #$22 0600 STA SDMCTL; ... SO WE'LL TURN ANTIC BACK ON NOW 0610 ; 0620 FINI 0630 RTS
Download / View (Assembly source code)
How It Works
We've already covered just about everything in this program, so it's probably fairly self-explanatory. However, if you're using an Atari Assembler cartridge or an Atari Macro Assembler and Text Editor package, you'll have one problem with the program: it won't work! That's because Atari's two assemblers, as sophisticated as they may be, they are not equipped with one handly little directive that the MAC/65 assembler does have, the .SBYTE directive used in lines 220 through 260 of our display list program.
The MAC/65's .SBYTE directive was designed to convert ATASCII code, which is whay your computer uses to store text in its memory, to a completely different code that's used to display characters on the screen. This latter code is called, appropriately enough, screen code. It wouldn't be difficult to convert ATASCII to screen code if there were a direct one to one correlation between the two codes. Unfortunately, there is on such one-to-one relationship. To translate from ATASCII code to screen code, you have to add 64 to some character codes, subtract 32 from others, and leave still others alone. Here's an ATASCII to screen code converstion table that shows just what the translation process involves:
Converting ATASCII Code To Screen Code
OPERATION NEEDED ATASCII VALUES FOR CONVERSION ______________ ________________ 0 to 31 Add 64 32 to 95 Subtract 32 96 to 127 None 128 to 159 Add 64 160 to 223 Subtract 32 224 to 255 None
An Automatic Conversion Routine
If your assembler doesn't have an .SBYTE function, there is an assembly language routine you can use to make the above conversions. I wrote it before there was any such thing as a MAC/65 assembler or an .SBYTE directive. It's a "quick and dirty" routine that was dreamed up in a hurry, and if you're a good assembly language programmer, you can probably write a routine will do the same job faster, more efficiently, or both. But this one works just fine, and it will work with a block of text of any size. This little conversion subroutine simply performs the calculations in the above table automatically. Before you can use it, however, you'll have to make a few minor alterations in the main display list program you typed a few moments ago. Here's what you'll have to do:
Three Modifications
- Convert the .SBYTE directives in lines 220 to 260 to .BYTE directives (or to DB directives, if you're using an Atari Macro Assembler).
-
Add one variable and one constant to the symbol table in your display list program.
The variable, which I'll call TEMPTR (for "temporary pointer"), will have to be located on page zero, since it will be used with indirect indexed addressing, an addressing mode that demands two zero page locations.
The constant you'll be using, called EOF, will have the literal value $88, which is the ATASCII code for an end of file character.
You can add these symbols to the symbol table in your title screen program with these lines:
65 TEMPTR = $CC 66 EOF = $88 67 ;
265 .BYTE EOF
Now Let's Get Started
The conversion routine starts off by storing the starting address of teh data for our new display list into a pair of pointers called TEMPTR and TEMPTR+1. Then, using indirect indexed addressing, it moves through the text to be converted character by character. Before it performs each conversion, however, it checks to see if the character in question is an end of file character ($88). If the character in question is an EOF character, the subroutine ends, since that means that all necessary conversion have been performed and that it's time to end the conversion routine. If the character is not an EOF character, the program goes ahead and performs the necessary conversion (if one is needed). Then it moves on to the next character.
Faking the .SBYTE Directive
If your assembler isn't equipped with an .SBYTE directive, or if, for some reason, you don't want to use the .SBYTE directive that the MAC/65 assembler provides, then you can use this conversion routine. Just type it into RAM, and then jump to it after your display list is loaded into memory but before the code that initilizes your display list begins. you can do that with this line:
475 JSR FIX
Here's the conversion program:
Note: Before assembling this program type SIZE to verify that MEMLO (the second number) is less than the starting address of the object code in line 40.
If it isn't, you should either change the starting address (e.g., 40 *=$5000), change LOMEM (e.g., LOMEM 4000), or remove comments from the source code so that MEMLO is less than the starting address.
An ATASCII-ASCII Conversion Subroutine
2000 ; 2010 ; FIX DATA 2020 ; 2030 FIX NOP 2040 LDA #START&255; STARTING ADDRESS OF NEW DISPLAY LIST (LOW BYTE) 2050 STA TEMPTR 2060 LDA #START/256; NEW DISPLAY LIST ADDRESS (HIGH BYTE) 2070 STA TEMPTR+1 2080 ; 2090 ; "FIX DATA" SUBROUTINE 2100 ; 2110 LDY #0; LOAD Y REGISTER WITH DUMMY 0 FOR INDIRECT INDEXED ADDRESSING 2120 FXDT NOP 2130 LDA (TEMPTR),Y; START WITH FIRST CHARACTER IN BLOCK 2140 CMP #EOF; IS IT AN END OF FILE CHARACTER ($88)? 2150 BEQ DONE; IF SO, WE'RE DONE -- EXIT SUBROUTINE 2160 JSR FXCH; ELSE JUMP TO "FIX CHARACTER" SUBROUTINE 2170 STA (TEMPTR), Y; THEN REPLACE OLD CHARACTER WITH NEW ONE 2180 INC TEMPTR; ... AND INCREMENT TEMPTR (LOW BYTE) 2190 BNE FXDT; IF NO CARRY TO HIGH BYTE, START AGAIN 2200 INC TEMPTR+1; ELSE INCREMENT TEMPTR'S HIGH BYTE 2210 JMP FXDT; ... AND THEN GO BACK TO FXDT AND START AGAIN 2220 ; 2230 DONE NOP; MAIN SUBROUTINE DONE -- ALL CHARACTERS CONVERTED 2240 RTS; SO NOW WE RETURN TO PROGRAM IN PROGRESS 2250 ; 2260 ; "FIX CHARACTER" ROUTINE" 2270 ; 2280 FXCH NOP 2290 CMP #32; IS CHARACTER CODE <32 ? 2300 BCC ADD64; IF SO, JUMP TO "ADD64" ROUTINE 2310 ; 2320 CMP #96; IS IT < 96? 2330 BCC SUB32; IF SO, JUMP TO "SUBTRACT 32" ROUTINE 2340 ; 2350 CMP #128; < 128? 2360 BCC FIXT; IF SO; JUMP TO "FIXT" ROUTINE (NO ACTION) 2370 ; 2380 CMP #160; < 160? 2390 BCC ADD64; IF SO, JUMP TO ADD64 2400 ; 2410 CMP #224; < 224? 2420 BCC SUB32; IF SO , JUMP TO SUB32 2430 ; 2440 JMP FIXT; IF BETWEEN 224 AND 255, NO ACTION NEEDED 2450 ; 2460 ADD64 NOP 2470 CLC; CLEAR CARRY TO ADD 2480 ADC #64; THEN ADD 64 2490 JMP FIXT; RETURN TO MAIN(FXDT) SUBROUTINE 2500 ; 2510 SUB32 NOP 2520 SEC; SET CARRY FOR SUBTRACTION 2530 SBC #32; AND SUBTRACT 32 2540 FIXT NOP 2550 RTS; RETURN TO MAIN (FIXDT) SUBROUTINE
Download / View (Assembly source code)
Coarse Scrolling
As soon as you've typed this program and savid it on a disk, you can run it, and if you've typed it exactly as written, you should have no problems. As soon as you have it up and running, you can start fixing it up so that it will be even fancier, with a technique known as scrolling. Before I start discussing scrolling, though, I'd like to suggest that you make on more small modification in the display list program that'vee been working on. We'll be scrolling just one line in the program - the line that says "THE BIG PROGRAM." And we'll need to insert some blank spaces before and after that line so that it will scroll across the screen properly. With these spaces inserted, the screen will start off with a blank space where the words "THE BIG PROGRAM" should be. Then these three words will come scrolling across the screen, like those ticker tape syle signs you sometimes see in store windows and on TV. To provide this new spacing, you'll have to change one line of your original display list program, and then add two morelines. Here are the threee lines we'll need (230 is the amended line, and 225 and 235 are the new ones):
225 LINE2 .SBYTE " " 230 .SBYTE " the big program " 235 .SBYTE " "
Once these lines are modified, it isn't difficult to implement a primitive sort of scrolling (called coarse scrolling) in our display list program. To implement a coarse scroll, all you have to do is set up a loop that keeps incrementing or decrementing certain addresses in a program - specifically, the addresses that follow the LMS instructions in a display list. It your scrolling program is written in assembly langauge, it will also have to include some sort of delay loop, since machine language is so fast that without some sort of delay, it will cause a scroll line to zoom by so rapidly that it turns into a blur.
Here is a display list and an accompanying display list implementation routine will add coarse scrolling to your title screen program. Make the following changes in the program, and the line that reads "THE BIG PROGRAM" will scroll across the screen over and over again in an endless loop. The text will move in a very jerky manner, one full letter at a time. But don't wory; in the next chapter, we'll smooth out that action with an assembly language technique know as fine scrolling.
An Example Of Coarse Scrolling
10 ; 20 ;HELLO SCREEN (COARSE) 30 ; 40 *=$3000 50 JMP INIT 60 ; 70 TCKPTR=$2000 80 ; 90 SDMCTL=$022F 100 ; 110 SDLSTL=$0230 120 SDLSTH=$0231 130 ; 140 COLOR0=$02C4; OS COLOR REGISTERS 150 COLOR1=$02C5 160 COLOR2=$02C6 170 COLOR3=$02C7 180 COLOR4=$02C8 190 ; 200 ;DISPLAY LIST DATA 210 ; 220 START 230 LINE1 .SBYTE " PRESENTING " 240 LINE2 .SBYTE " " 250 .SBYTE " THE BIG PROGRAM " 260 .SBYTE " " 270 LINE3 .SBYTE " By (You" 280 .SBYTE "r Name) " 290 LINE4 .SBYTE " PLEASE STAND BY" 300 ; 310 ; HELLO DISPLAY LIST 320 ; 330 HLIST 340 .BYTE $70,$70,$70 350 .BYTE $70, $70, $70, $70,$70 360 .BYTE $46 370 .WORD LINE1 380 .BYTE $70,$70,$70,$70,$47 390 SCROLN NOP 400 .WORD $00 410 .BYTE $70,$42 420 .WORD LINE3 430 .BYTE $70,$70,$70,$70,$46 440 .WORD LINE4 450 .BYTE $70,$70,$70,$70,$70 460 .BYTE $41 470 .WORD HLIST 480 ; 490 ;RUN PROGRAM 500 ; 510 INIT NOP 520 LDA COLOR3 530 STA COLOR1 540 LDA COLOR4 550 STA COLOR2 560 ; 570 LDA #0 580 STA SDMCTL 590 LDA #HLIST&255 600 STA SDLSTL 610 LDA # HLIST/256 620 STA SDLSTH 630 LDA #$22 640 STA SDMCTL 650 ; 660 ; COARSE SCROLLING ROUTINE 670 ; 680 LDA #40 690 STA TCKPTR 700 JSR TCKSET 710 ; 720 COARSE NOP 730 LDY TCKPTR; 40 TO START 740 DEY 750 BNE SCORSE 760 LDY #40 770 JSR TCKSET 780 SCORSE NOP 790 STY TCKPTR 800 INC SCROLN 810 BNE LEAP 820 INC SCROLN+1 830 ; 840 ; DELAY LOOP 850 ; 860 LEAP NOP 870 TYA 880 PHA; SAVE Y REGISTER 890 LDX #$FF 900 XLOOP NOP 910 LDY #$80 920 YLOOP NOP 930 DEY 940 BNE YLOOP 950 ; 960 DEX 970 BNE XLOOP 980 PLA 990 TAY; RESTORE Y REG 1000 ; 1010 JMP COARSE 1020 ; 1030 TCKSET NOP 1040 LDA #LINE2&255 1050 STA SCROLN 1060 LDA #LINE2/256 1070 STA SCROLN+1 1080 ENDIT NOP 1090 RTS
Download / View (Assembly source code)
If you type the above modifications into your display list program and run it, what you'll see is an example of coarse scrolling. The line that reads "THE BIG PROGRAM" will come jumping across the screen, a letter at a time , in a jerky kind of way that could get rather disconcerting if you had to look at it for very long. Coarse scrolling is bad enough when it's used to move just one line across a screen. But it becomes even more nerve shattering when it's used to scroll an entire screen display.
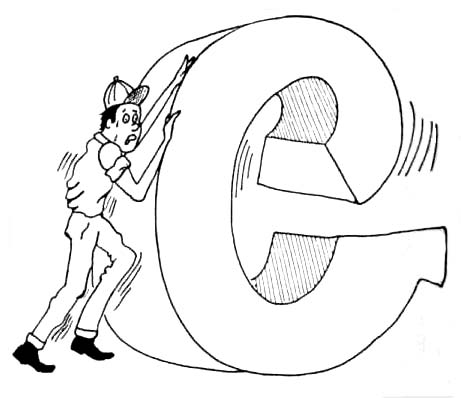
To scroll more than one line on your screen, up to and including the maximum number of lines in a full screen display, all you have to do is go to your display list and insert an LMS instruction before every line you want scrolled. If you want to scroll you entire screen, you can precede every line with an LMS instruction! Then, to scroll your screen horizontally, all you'll have to do is set up a loop that progressively increments or decrements the starting address of the data that appears on every line. If you increment those address, your display will scroll from right to left. If yfouf decrement them, it will scroll from left to right.
It's just as easy to do corase vertical scrolling as it is to do coarse horizontal scrolling. To scroll a screen display vertically, all you have to do is increment or decrement the LMS address of each line by the number of characters in the lines being scrolled, instead of by just one character at a time. When you set up this kind of scrolling action, you have to count the number of characters in each line very carefully, so your characters won't move back and forth on the screen as they scroll up or down. Coarse vertical scrolling, like coarse horizontal scrolling, can be used to scroll any number of lines on a monitor screen. Whether you're aware of it or not, in fact you've probably enountered coarse vertical scrolling in action many times. It's the kind of scrolling you see when you're writing a BASIC or assembly language program, reach the bottom line on your monitor screen, and hit a carriage return. When you do that, your entire screen display moves up one line, using a corase vertical scrolling routine.
Coarse scrolling is fine when it's used that way, just one line at a time, but it doesn't work very well in more demanding applications, such as moving screen displays around in arcade style games. Unfortunately for BASIC programmers, coarse scrolling is the only kind that Atari BASIC supports. To do smooth scrolling, you have to use - you guessed it! - assembly language. In the next (and last) chapter of this book, you'll learn how to use smooth scrolling in assembly language programs. And, as if that weren't enough, you'll also learn how to create and animate characters sets, how to use player-missile graphics, and how to write assembly language programs that call for the use of game controllers.
Return to Table of Contents | Previous Chapter | Next Chapter