
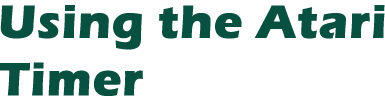
Have you ever written a program and wanted a specific time delay? What did you do? Some of us figured a FOR/NEXT loop was the answer, so we set to work with our stopwatches until we found that the following takes about three seconds to write "STOP":
10 PRINT "BEGIN" 20 FOR X=1 TO 1000 30 NEXT X 40 PRINT "STOP"
Then we went along and wrote our programs and found that our three-second delay had become five, six, or even ten seconds. Why? Because the Atari FOR/NEXT loops take longer as you add lines of code to the program.
There is a better way. Yes, machine language routines are great for timing on the Atari, especially if you know how to use locations 536 to 558 ($218 to $22E). But it can be most disconcerting if you allow some of those registers to drop to zero unchecked.
BASIC programmers, there is a way. Use memory locations 18, 19, and 20.
These timers work like the mileage guage on a car's speedometer: one counts up and then sets the one next to it which, in turn, sets the next one. Each counter on the speedometer goes up when the one to its right hits ten. In the computer, they count up to 255 before going back to zero.
Register number 20 counts at the rate of 60 numbers per second up to number 255, then increments register 19 by one and starts over. When register 19 reaches 255, it increments register 18 by one. If you POKE zero into all three registers, it will take about 1092 seconds before a one appears in register 18 (more than 18 minutes). The table gives some times (it assumes all three registers began with zero). Notice that it would take more than 77 hours for memory location 18 to reach 255.
Well, how does this all help? Let's look at our short program again. We can rewrite it this way:
10 PRINT "BEGIN": POKE 20,0 20 IF PEEK(20)<180 THEN 20 30 PRINT "STOP"
This routine will continue to take three seconds no matter how long your program. Well, not exactly; since it is written in BASIC, the longer the program, the longer the routine will take. But the invluence of the program length will usually be negligible.
Included here are three programs which demonstrate a much more functional use of this timer. Type in Program 1, leaving out the REM statements. This program tells the user the time interval between the pressing of RETURN after typing RUN and the pressing of RETURN a second time. Notice that if you press another key the computer goes back to line 140.
This short program demonstrates several useful concepts. First, the computer is looking for a particular input, in this case the RETURN key (ATASCII 155). Second, line 160 PEEKs at registers 18, 19, and 20. Notice we POKEd location 20 last on line 130 and PEEKed it first on line 160. Third, line 170 contains the important formula for converting the invormation in locations 18, 19, and 20 to seconds. Why 4.267? Because 256 divided by 60 numbers per second equals 4.267. Fourth, lines 180 to 200 convert the total number of seconds to minutes and seconds.
Program 2 is a bit more useful. It is a timed math quiz in which the user is allowed eight and one-half seconds to answer. Line 140 is used to check if a key has been pressed. If no key has been pressed, then the program goes back to check how much time has elapsed. Once a key is pressed, the computer GETs the ATASCII code and calls it A1. At lines 160 and 170, A1 is converted to its CHR$ and placed in its proper place in ANS$. If A1 equals 155 (ATASCII code for the RETURN key), the program moves to line 220, where the value of ANS$ is put into variable ANS.
The final illustration, Program 3, is also a math quiz. In this case the user is given unlimited time. The program combines elements of both Programs 1 and 2.
This Atari timing device should be beneficial whether you wish to impose a time limit, simply time answers, or have users compete against each other or themselves. The timer has applications for both educational programming and games. With some experimentation you should be able to adapt this timing device for use with your own programs.
LOC.20 |
LOC.20 |
LOC.20 |
TIME MIN:SEC |
60 | 0 | 0 | 0:01 |
60 | 1 | 0 | 0:05 |
0 | 2 | 0 | 0:08 |
100 | 2 | 0 | 0:10 |
0 | 3 | 0 | 0:12 |
100 | 4 | 0 | 0:18 |
21 | 14 | 0 | 1:00 |
42 | 28 | 0 | 2:00 |
84 | 56 | 0 | 4:00 |
176 | 112 | 0 | 8:00 |
0 | 255 | 0 | 18:08 |
0 | 60 | 2 | 40:40 |
0 | 0 | 16 | 291:17 |
0 | 0 | 100 | 1820:35 |
0 | 0 | 150 | 2730:52 |
0 | 0 | 255 | 4642:29 |
10 REM ATARI TIMER 20 REM 30 REM THIS PROGRAM DEMONSTRATES HOW 40 REM TO USE ATARI TIMER: 50 REM ADDRESS 18,19,20 60 REM IT FIGURES HOW LONG IT TAKES 70 REM YOU TO PRESS THE <RETURN> KEY 80 REM RUN THE PROGRAM THEN PRESS 90 REM <RETURN> 100 REM PROGRAM RUNS BETTER WITHOUT 110 REM REMARK STATEMENTS OR GOTO 120 120 OPEN #1,4,0,"K:" 130 FOR Z=18 TO 20:POKE Z,0:NEXT Z 140 GET #1,D:IF D=155 THEN 160 150 GOTO 140 160 A=PEEK(20):B=PEEK(19):C=PEEK(18) 170 SEC=INT((4.267*256*C)+(B*4.267)+(A/60)) 180 MIN=INT(SEC/60) 190 M=MIN*60 200 SEC=SEC-M 210 PRINT MIN;" MINUTES ";SEC;" SECONDS"
Listing. Atari Timer.
With REM statements: | Download (Saved BASIC) / Download (Listed BASIC) |
Without REM statements: | Download (Saved BASIC) / Download (Listed BASIC) |
10 REM TIMED MATH QUIZ 20 REM 30 REM THIS IS A TIMED MATH QUIZ 40 REM CHANGE LINE 130 TO A=1 50 REM ALLOWS 4 1/4 SECOND 60 REM A=2 ALLOWS 8 1/2 SECONDS 70 REM A=3 ALLOWS 12 3/4 SECONDS, ETC. 80 OPEN #1,4,0,"K:":DIM ANS$(10) 90 PRINT :Q1=INT(RND(0)*20):Q2=INT(RND(0)*20):X=1 100 PRINT Q1;" + ";Q2;"="; 110 POKE 18,0:POKE 19,0:POKE 20,0 120 A=PEEK(19):B=PEEK(20) 130 IF A=2 THEN 180:REM 8 1/2 SECONDS 140 IF PEEK(764)=255 THEN 120 150 GET #1,A1:IF A1=155 THEN 220 160 ANS$(X,X)=CHR$(A1) 170 PRINT ANS$(X,X);:X=X+1:GOTO 120 180 PRINT :PRINT "TIME'S UP" 190 PRINT "THE ANSWER IS ";Q1+Q2 200 FOR W=1 TO 400:NEXT W 210 ANS$=" ":GOTO 90 220 ANS=VAL(ANS$):PRINT 230 IF ANS=Q1+Q2 THEN PRINT :PRINT "CORRECT":GOTO 200 240 PRINT :PRINT "SORRY":PRINT :GOTO 190
Listing. Timed Math Quiz.
With REM statements: | Download (Saved BASIC) / Download (Listed BASIC) |
Without REM statements: | Download (Saved BASIC) / Download (Listed BASIC) |
10 REM REVISED MATH QUIZ 20 REM 30 REM THIS PROGRAM COMBINES ELEMENTS 40 REM OF PROGRAMS 1 AND 2. 50 REM IT GIVES MATH QUIZ AND TELL HOW 60 REM LONG IT TOOK YOU TO DO EACH 70 REM PROBLEM. 80 OPEN #1,4,0,"K:":DIM ANS$(10) 90 PRINT :Q1=INT(RND(0)*20):Q2=INT(RND(0)*20):X=1 100 PRINT Q1;" + ";Q2;"="; 110 POKE 18,0:POKE 19,0:POKE 20,0 120 IF PEEK(764)=255 THEN 120 130 GET #1,A1:IF A1=155 THEN 190 140 ANS$(X,X)=CHR$(A1) 150 PRINT ANS$(X,X);:X=X+1:GOTO 120 160 PRINT "THE ANSWER IS ";Q1+Q2 170 FOR W=1 TO 1000:NEXT W 180 ANS$=" ":GOTO 90 190 A=PEEK(20):B=PEEK(19):C=PEEK(18) 200 ANS=VAL(ANS$):PRINT 210 IF ANS=Q1+Q2 THEN PRINT :PRINT "CORRECT":GOTO 230 220 PRINT :PRINT "SORRY" 230 SEC=INT((4.25*256*C)+(B*4.25)+(A/60)) 240 MIN=INT(SEC/60) 250 M=MIN*60 260 SEC=SEC-M 270 IF MIN<>0 THEN 290 280 PRINT "THAT TOOK YOU ";SEC;" SECONDS":GOTO 300 290 PRINT "THAT TOOK YOU ";MIN;" MINUTES":PRINT "AND ";SEC;" SECONDS" 300 GOTO 170
Listing. Revised Math Quiz.
With REM statements: | Download (Saved BASIC) / Download (Listed BASIC) |
Without REM statements: | Download (Saved BASIC) / Download (Listed BASIC) |
Return to Table of Contents | Previous Section | Next Section